class: left, bottom background-image: url("images/contour.png") background-position: right background-size: auto # Factor Data Type ### Categorical Data, Ordered & Unordered <p> </p> <p> </p> <img src="images/logo1.svg" width="400px"> --- class: sectionTitle # Categorical Data --- background-image: url("https://live.staticflickr.com/65535/51479744159_93f1660faf_o_d.jpg") background-position: center background-size: cover --- # Making Some Categorical Data Types .left-column[ Unique and individual groupsing that can be applied to a study design. - Case sensitive - Can be ordinal - Typically defined as `character` type ] .right-column[ ```r weekdays <- c("Monday","Tuesday","Wednesday", "Thursday","Friday","Saturday", "Sunday") class( weekdays ) ``` ``` ## [1] "character" ``` ```r weekdays ``` ``` ## [1] "Monday" "Tuesday" "Wednesday" "Thursday" "Friday" "Saturday" ## [7] "Sunday" ``` ] --- # Making Up Some Data 🤷🏻 The function `sample()` allows us to take a random sample of elements from a vector of potential values. ```r chooseOne <- sample( c("Heads","Tails"), size=1 ) chooseOne ``` ``` ## [1] "Tails" ``` -- However, if we want a large number items, we can have them with or without replacement. ```r sample( c("Heads","Tails"), size=10, replace=TRUE ) ``` ``` ## [1] "Heads" "Heads" "Tails" "Tails" "Tails" "Tails" "Heads" "Heads" "Tails" ## [10] "Tails" ``` --- # Weekdays as example We'll pretend we have a bunch of data related to the day of the week. ```r days <- sample( weekdays, size=40, replace=TRUE) summary( days ) ``` ``` ## Length Class Mode ## 40 character character ``` ```r days ``` ``` ## [1] "Wednesday" "Wednesday" "Wednesday" "Wednesday" "Saturday" "Friday" ## [7] "Wednesday" "Monday" "Saturday" "Monday" "Monday" "Monday" ## [13] "Thursday" "Sunday" "Friday" "Wednesday" "Monday" "Thursday" ## [19] "Sunday" "Tuesday" "Saturday" "Monday" "Wednesday" "Saturday" ## [25] "Friday" "Wednesday" "Monday" "Monday" "Thursday" "Friday" ## [31] "Tuesday" "Thursday" "Friday" "Monday" "Monday" "Wednesday" ## [37] "Wednesday" "Friday" "Thursday" "Sunday" ``` --- # Turn it into a `factor` ```r data <- factor( days ) is.factor( data ) ``` ``` ## [1] TRUE ``` ```r class( data ) ``` ``` ## [1] "factor" ``` --- # Data Type Specific Printing & Summaries ```r data ``` ``` ## [1] Wednesday Wednesday Wednesday Wednesday Saturday Friday Wednesday ## [8] Monday Saturday Monday Monday Monday Thursday Sunday ## [15] Friday Wednesday Monday Thursday Sunday Tuesday Saturday ## [22] Monday Wednesday Saturday Friday Wednesday Monday Monday ## [29] Thursday Friday Tuesday Thursday Friday Monday Monday ## [36] Wednesday Wednesday Friday Thursday Sunday ## Levels: Friday Monday Saturday Sunday Thursday Tuesday Wednesday ``` -- ```r summary( data ) ``` ``` ## Friday Monday Saturday Sunday Thursday Tuesday Wednesday ## 6 10 4 3 5 2 10 ``` --- # Factor Levels Each factor variable is defined by the `levels` that constitute the data. This is a .red[finite] set of unique values ```r levels( data) ``` ``` ## [1] "Friday" "Monday" "Saturday" "Sunday" "Thursday" "Tuesday" ## [7] "Wednesday" ``` --- # Factors Ordination .pull-left[ Examples of conditions where ordination matters: - Fertilizer Treatments in KG of N<sub>2</sub> per hectare: 10 kg N<sub>2</sub>, 20 N<sub>2</sub>, 30 N<sub>2</sub>, - Days of the Week: `Friday` is not followed by `Monday`, - Life History Stage: seed, seedling, juvenile, adult, etc. ] .pull-right[ Examples where Ordination is Irrelevant - River - State or Region - Sample Location ] --- # Factor Ordination If a factor is ordinal, it allows us to use relational comparison operators. ```r data[1] < data[2] ``` .red[\# Warning message: ] .red[ In Ops.factor(data[1], data[2]) : '<' not meaningful for factors] -- ```r is.ordered(data) ``` ``` ## [1] FALSE ``` --- # Making Ordered Factors To make them just ordered. ```r data <- factor( days, ordered = TRUE) is.ordered( data ) ``` ``` ## [1] TRUE ``` -- ```r data ``` ``` ## [1] Wednesday Wednesday Wednesday Wednesday Saturday Friday Wednesday ## [8] Monday Saturday Monday Monday Monday Thursday Sunday ## [15] Friday Wednesday Monday Thursday Sunday Tuesday Saturday ## [22] Monday Wednesday Saturday Friday Wednesday Monday Monday ## [29] Thursday Friday Tuesday Thursday Friday Monday Monday ## [36] Wednesday Wednesday Friday Thursday Sunday ## 7 Levels: Friday < Monday < Saturday < Sunday < Thursday < ... < Wednesday ``` .red[Problem:] it orders things in *alphabetical* order. --- # Specifying the Order of Ordinal Factors ```r data <- factor( days, ordered = TRUE, levels = weekdays) data ``` ``` ## [1] Wednesday Wednesday Wednesday Wednesday Saturday Friday Wednesday ## [8] Monday Saturday Monday Monday Monday Thursday Sunday ## [15] Friday Wednesday Monday Thursday Sunday Tuesday Saturday ## [22] Monday Wednesday Saturday Friday Wednesday Monday Monday ## [29] Thursday Friday Tuesday Thursday Friday Monday Monday ## [36] Wednesday Wednesday Friday Thursday Sunday ## 7 Levels: Monday < Tuesday < Wednesday < Thursday < Friday < ... < Sunday ``` -- <center><img src="https://media.giphy.com/media/KEYbcgR8oKQzwpwvLU/giphy-downsized-large.gif?cid=ecf05e47pagn8g10iwyysc86x07tgew8evhr1dbkahub7cuu&rid=giphy-downsized-large.gif&ct=g" height=200></center> --- # Sorting ```r sort( data ) ``` ``` ## [1] Monday Monday Monday Monday Monday Monday Monday ## [8] Monday Monday Monday Tuesday Tuesday Wednesday Wednesday ## [15] Wednesday Wednesday Wednesday Wednesday Wednesday Wednesday Wednesday ## [22] Wednesday Thursday Thursday Thursday Thursday Thursday Friday ## [29] Friday Friday Friday Friday Friday Saturday Saturday ## [36] Saturday Saturday Sunday Sunday Sunday ## 7 Levels: Monday < Tuesday < Wednesday < Thursday < Friday < ... < Sunday ``` --- # Fixed Set of States ```r data[3] <- "Bob" data ``` ``` ## [1] Wednesday Wednesday <NA> Wednesday Saturday Friday Wednesday ## [8] Monday Saturday Monday Monday Monday Thursday Sunday ## [15] Friday Wednesday Monday Thursday Sunday Tuesday Saturday ## [22] Monday Wednesday Saturday Friday Wednesday Monday Monday ## [29] Thursday Friday Tuesday Thursday Friday Monday Monday ## [36] Wednesday Wednesday Friday Thursday Sunday ## 7 Levels: Monday < Tuesday < Wednesday < Thursday < Friday < ... < Sunday ``` --- class: sectionTitle # forcats ### rep(
, times = 4) --- # The `forcats` library Part of the `tidyverse` group of packages. ```r library(forcats) ``` This library has a lot of helper functions that make working with factors a bit easier. I'm going to give you a few examples here but .red[strongly] encourage you to look a the cheat sheet for all the other options. --- # Counting Factors ```r fct_count( data ) ``` ``` ## # A tibble: 8 × 2 ## f n ## <fct> <int> ## 1 Monday 10 ## 2 Tuesday 2 ## 3 Wednesday 9 ## 4 Thursday 5 ## 5 Friday 6 ## 6 Saturday 4 ## 7 Sunday 3 ## 8 <NA> 1 ``` --- # Lumping Factors ```r lumped <- fct_lump_min( data, min = 5) fct_count( lumped ) ``` ``` ## # A tibble: 6 × 2 ## f n ## <fct> <int> ## 1 Monday 10 ## 2 Wednesday 9 ## 3 Thursday 5 ## 4 Friday 6 ## 5 Other 9 ## 6 <NA> 1 ``` --- # Reordering Factors We can reorder by *appearance order*, *observations*, or *numeric* *By Frequency* ```r freq <- fct_infreq( data ) levels( freq ) ``` ``` ## [1] "Monday" "Wednesday" "Friday" "Thursday" "Saturday" "Sunday" ## [7] "Tuesday" ``` *By Order of Appearance* ```r ordered <- fct_inorder( data ) levels( ordered ) ``` ``` ## [1] "Wednesday" "Saturday" "Friday" "Monday" "Thursday" "Sunday" ## [7] "Tuesday" ``` --- # Reorder Specific Levels ```r newWeek <- fct_relevel( data, "Saturday", "Sunday") levels( newWeek ) ``` ``` ## [1] "Saturday" "Sunday" "Monday" "Tuesday" "Wednesday" "Thursday" ## [7] "Friday" ``` --- # Dropping Missing Levels ```r data <- sample( weekdays[1:5], size=40, replace=TRUE ) data <- factor( data, ordered=TRUE, levels = weekdays ) summary( data ) ``` ``` ## Monday Tuesday Wednesday Thursday Friday Saturday Sunday ## 9 8 9 7 7 0 0 ``` -- ```r fct_drop( data ) -> dropped summary( dropped ) ``` ``` ## Monday Tuesday Wednesday Thursday Friday ## 9 8 9 7 7 ``` --- class: sectionTitle # Fancy Tables ### `knitr` + `table` = `kable` --- # Fancy Tables `kable` We've already learned how to make tables by hand using `---|---` and such, however, it may be important to work with `data.frame` and `tibble` objects and allow them to become nicely presented tables. ```r library( knitr ) ``` The function `knitr::kable()` will take a data frame and output a wide variety of tabular data. To do this, the essence is that we need to make a data frame whose rows and columns are exactly what we'd like to have translated into markdown and presented. --- # Example data `iris` .pull-left[  ] .pull-right[ ## Ronald Aylmer Fisher 1890 - 1962 British polymath, mathematician, statistican, geneticist, and academic. Founded things such as: - Fundamental Theorem of Natural Selection, - The `F` test, - The `Exact` test, - Linear Discriminant Analysis, - Inverse probability - Intra-class correlations - Sexy son hypothesis.... 🥰 ] --- # Iris ```r head( iris ) ``` ``` ## Sepal.Length Sepal.Width Petal.Length Petal.Width Species ## 1 5.1 3.5 1.4 0.2 setosa ## 2 4.9 3.0 1.4 0.2 setosa ## 3 4.7 3.2 1.3 0.2 setosa ## 4 4.6 3.1 1.5 0.2 setosa ## 5 5.0 3.6 1.4 0.2 setosa ## 6 5.4 3.9 1.7 0.4 setosa ``` -- ```r summary( iris ) ``` ``` ## Sepal.Length Sepal.Width Petal.Length Petal.Width ## Min. :4.300 Min. :2.000 Min. :1.000 Min. :0.100 ## 1st Qu.:5.100 1st Qu.:2.800 1st Qu.:1.600 1st Qu.:0.300 ## Median :5.800 Median :3.000 Median :4.350 Median :1.300 ## Mean :5.843 Mean :3.057 Mean :3.758 Mean :1.199 ## 3rd Qu.:6.400 3rd Qu.:3.300 3rd Qu.:5.100 3rd Qu.:1.800 ## Max. :7.900 Max. :4.400 Max. :6.900 Max. :2.500 ## Species ## setosa :50 ## versicolor:50 ## virginica :50 ## ## ## ``` --- # Factors and `by()` One common thing to use factors for is to grab a group of data and perform an operation on them. **Question: What is mean and variance in sepal length for the three iris species.** -- The general form of the `by()` function. ```r by( data, index, function) ``` --- ```r by( iris$Sepal.Length, iris$Species, mean ) -> meanLength meanLength ``` ``` ## iris$Species: setosa ## [1] 5.006 ## ------------------------------------------------------------ ## iris$Species: versicolor ## [1] 5.936 ## ------------------------------------------------------------ ## iris$Species: virginica ## [1] 6.588 ``` -- ```r by( iris$Sepal.Length, iris$Species, var ) -> varLength varLength ``` ``` ## iris$Species: setosa ## [1] 0.124249 ## ------------------------------------------------------------ ## iris$Species: versicolor ## [1] 0.2664327 ## ------------------------------------------------------------ ## iris$Species: virginica ## [1] 0.4043429 ``` --- # Missing Data .pull-left[ Missing data is a .red[fact of life] and `R` is very opinionated about how it handles missing values. In general, missing data is encoded as `NA` and is a valid entry for any data type (character, numeric, logical, factor, etc.). Where this becomes tricky is when we are doing operations on data that has missing values. `R` could take two routes: 1. It could ignore the data and give you the answer directly as if the data were not missing, or 2. It could let you know that there is missing data and make you do something about it. Fortunately, `R` took the second route. ] -- .pull-right[ An example from the iris data, I'm going to add some missing data to it. ```r df <- iris[, 4:5] df$Petal.Width[ c(2,6,12) ] <- NA summary( df) ``` ``` ## Petal.Width Species ## Min. :0.100 setosa :50 ## 1st Qu.:0.300 versicolor:50 ## Median :1.300 virginica :50 ## Mean :1.218 ## 3rd Qu.:1.800 ## Max. :2.500 ## NA's :3 ``` Notice how the missing data is denoted in the summary. ] --- # Missing Data .pull-left[ ### Indications of Missing Data When we perform a mathematical or statistical operation on data that has missing elements `R` will **always** return NA as the result. ```r mean( df$Petal.Width ) ``` ``` ## [1] NA ``` This warns you that .red[at least one] of the observations in the data is missing. Same output for using `by()`, it will put `NA` into each level that has at least one missing value. ```r by( df$Petal.Width, df$Species, mean ) ``` ``` ## df$Species: setosa ## [1] NA ## ------------------------------------------------------------ ## df$Species: versicolor ## [1] 1.326 ## ------------------------------------------------------------ ## df$Species: virginica ## [1] 2.026 ``` ] -- .pull-right[ ### Working with Missing Data To acknowledge that there are missing data and you still want the values, you need to tell the function you are using that data is missing and you are OK with that using the optional argument `na.rm=TRUE` (`na` = missing data & `rm` is *remove*). ```r mean( df$Petal.Width, na.rm=TRUE) ``` ``` ## [1] 1.218367 ``` To pass this to the `by()` function, we add the optional argument `na.rm=TRUE` and `by()` passes it along to the `mean` function as "..." ```r by( df$Petal.Width, df$Species, mean, na.rm=TRUE ) ``` ``` ## df$Species: setosa ## [1] 0.2446809 ## ------------------------------------------------------------ ## df$Species: versicolor ## [1] 1.326 ## ------------------------------------------------------------ ## df$Species: virginica ## [1] 2.026 ``` ] --- # Creating a Data Frame ```r library( tidyverse ) df <- tibble( Species = levels( iris$Species ), Average = meanLength, Variance = varLength ) df ``` ``` ## # A tibble: 3 × 3 ## Species Average Variance ## <chr> <by> <by> ## 1 setosa 5.006 0.1242490 ## 2 versicolor 5.936 0.2664327 ## 3 virginica 6.588 0.4043429 ``` --- # A Basic Table Using `kable` ```r kable(df) ``` <table> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Adding A Caption to the table ```r irisTable <- kable( df, caption = "Mean and variance in sepal length for three iris species." ) irisTable ``` <table> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Even Fancier Stuff with `kableExtras` This library contains a bunch of additional helper functions that take a `kable` object and make it look better. ```r library( kableExtra) ``` You must go check out [this](https://cran.r-project.org/web/packages/kableExtra/vignettes/awesome_table_in_html.html) webpage (which is an RMarkdown page by the way) to see all the other ways you can fancy up your tables. --- # Table Themes ```r kable_paper( irisTable ) ``` <table class=" lightable-paper" style='font-family: "Arial Narrow", arial, helvetica, sans-serif; margin-left: auto; margin-right: auto;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Table Themes ```r kable_classic( irisTable ) ``` <table class=" lightable-classic" style='font-family: "Arial Narrow", "Source Sans Pro", sans-serif; margin-left: auto; margin-right: auto;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Table Themes ```r kable_classic_2( irisTable ) ``` <table class=" lightable-classic-2" style='font-family: "Arial Narrow", "Source Sans Pro", sans-serif; margin-left: auto; margin-right: auto;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Table Themes ```r kable_minimal( irisTable ) ``` <table class=" lightable-minimal" style='font-family: "Trebuchet MS", verdana, sans-serif; margin-left: auto; margin-right: auto;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Table Themes ```r kable_material( irisTable,lightable_options = c("striped", "hover") ) ``` <table class=" lightable-material lightable-striped lightable-hover" style='font-family: "Source Sans Pro", helvetica, sans-serif; margin-left: auto; margin-right: auto;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Table Themes ```r kable_material_dark( irisTable ) ``` <table class=" lightable-material-dark" style='font-family: "Source Sans Pro", helvetica, sans-serif; margin-left: auto; margin-right: auto;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Table Sizes ```r kable_paper(irisTable, full_width = FALSE ) ``` <table class=" lightable-paper" style='font-family: "Arial Narrow", arial, helvetica, sans-serif; width: auto !important; margin-left: auto; margin-right: auto;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Table Positions ```r kable_paper( irisTable, full_width=FALSE, position="right") ``` <table class=" lightable-paper" style='font-family: "Arial Narrow", arial, helvetica, sans-serif; width: auto !important; margin-right: 0; margin-left: auto'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- # Floating Tables - `position = "float_right"` Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut blandit libero sit amet porta elementum. In imperdiet tellus non odio porttitor auctor ac sit amet diam. Suspendisse eleifend vel nisi nec efficitur. Ut varius urna lectus, ac iaculis velit bibendum eget. Curabitur dignissim magna eu odio sagittis blandit. Vivamus sed ipsum mi. Etiam est leo, mollis ultrices dolor eget, consectetur euismod augue. In hac habitasse platea dictumst. Integer blandit ante magna, quis volutpat velit varius hendrerit. Vestibulum sit amet lacinia magna. Sed at varius nisl. Donec eu porta tellus, vitae rhoncus velit. <table class=" lightable-paper" style='font-family: "Arial Narrow", arial, helvetica, sans-serif; width: auto !important; float: right; margin-left: 10px;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> Maecenas euismod mattis neque. Ut at sapien lacinia, vehicula felis vitae, laoreet odio. Cras ut magna sed sapien scelerisque auctor maximus tincidunt arcu. Praesent vel accumsan leo. Etiam tempor leo placerat, commodo ante eu, posuere ligula. Sed purus justo, feugiat vel volutpat in, faucibus quis sem. Vivamus enim lacus, ultrices id erat in, posuere fringilla est. Nulla porttitor ac nunc nec efficitur. Duis tincidunt metus leo, at lacinia orci tristique in. Nulla nec elementum nibh, quis congue augue. Vivamus fermentum nec mauris nec vehicula. Proin laoreet sapien quis orci mollis, et condimentum ante tempor. Vivamus hendrerit ut sem a iaculis. Quisque mauris enim, accumsan sit amet fermentum quis, convallis a nisl. Donec elit orci, consectetur id vestibulum in, elementum nec magna. In lobortis erat velit. Nam sit amet finibus arcu. --- background-image: url("https://live.staticflickr.com/65535/51478614622_7b6fd7fe08_c_d.jpg") background-position: center background-size: contain --- # Even Fancier Grouped Headers ```r classic <- kable_paper( irisTable ) add_header_above( classic, c(" " = 1, "Sepal Length (cm)" = 2)) ``` <table class=" lightable-paper" style='font-family: "Arial Narrow", arial, helvetica, sans-serif; margin-left: auto; margin-right: auto;'> <caption>Mean and variance in sepal length for three iris species.</caption> <thead> <tr> <th style="empty-cells: hide;" colspan="1"></th> <th style="padding-bottom:0; padding-left:3px;padding-right:3px;text-align: center; " colspan="2"><div style="border-bottom: 1px solid #00000020; padding-bottom: 5px; ">Sepal Length (cm)</div></th> </tr> <tr> <th style="text-align:left;"> Species </th> <th style="text-align:right;"> Average </th> <th style="text-align:right;"> Variance </th> </tr> </thead> <tbody> <tr> <td style="text-align:left;"> setosa </td> <td style="text-align:right;"> 5.006 </td> <td style="text-align:right;"> 0.1242490 </td> </tr> <tr> <td style="text-align:left;"> versicolor </td> <td style="text-align:right;"> 5.936 </td> <td style="text-align:right;"> 0.2664327 </td> </tr> <tr> <td style="text-align:left;"> virginica </td> <td style="text-align:right;"> 6.588 </td> <td style="text-align:right;"> 0.4043429 </td> </tr> </tbody> </table> --- class: middle background-image: url("images/contour.png") background-position: right background-size: auto .center[ # Questions? 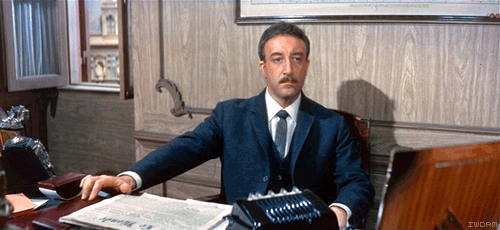 ] <p> </p> .bottom[ If you have any questions for about the content presented herein, please feel free to [submit them to me](https://docs.google.com/forms/d/e/1FAIpQLScrAGM5Zl8vZTPqV8DVSnSrf_5enypyp0717jG4PZiTlVHDjQ/viewform?usp=sf_link) and I'll get back to you as soon as possible.]