class: left, bottom background-image: url("images/contour.png") background-position: right background-size: auto # Numerical Data in R ### Environmental Data Literacy <p> </p> <p> </p> <img src="images/logo1.svg" width="400px"> --- # Numerical Data In `R`, numerical data is largely represented by a data type called `numeric`. -- - For most purposes, this is the only data type we will need (though `integer` types and specialized libraries exist). -- - Magnitude determined by your computer (my MacBook can handle 2.225074e-308 - 1.797693e+308). --- # Operators In many ways, `R` can act just like an interactive calculator. *Arithmetic operators* are just like normal. ```r x <- 10 y <- 23 x + y x - y x * y x / y ``` --- # Exponential Operators *Exponents* use the carat on the keyboard (on us-english keyboards it is above the #6 key). So the value of `\(2^16\)` is ```r 2^16 ``` ``` ## [1] 65536 ``` -- Roots are found by inverting the exponent. For example, the `\(\;^3\sqrt{27}\)` (cube-root of 27) is ```r 27^(1/3) ``` ``` ## [1] 3 ``` --- # Logrithms The logrithms are provided as the function `log()` which defaults to the natural log ```r log( 10 ) ``` ``` ## [1] 2.302585 ``` -- You can change the base by passing the function the optional argument (make sure you separate the value from the optional argument with a comma). ```r log( 10, base=10 ) ``` ``` ## [1] 1 ``` --- # Additional Operators .center[ *Potential Operations >>> Symbols on Keyboard* ] -- .red[ Live keyboard Demo ] --- # Additional Operators .center[ *Potential Operations >>> Symbols on Keyboard* ] *Modulus Operator* ```r 23 %% 10 ``` ``` ## [1] 3 ``` --- # Order of Operations The order of precedence for operations are just like you learned in math class. ```r x1 <- 23 y1 <- 55 x2 <- 56 y2 <- 63 distance <- sqrt( (x1-x2)^2 + (y1-y2)^2 ) distance ``` ``` ## [1] 33.95585 ``` --- # `?Syntax` Operator | Description ----------------|------------------------------------------- :: ::: | access variables in a namespace $ @ | component / slot extraction [ [[ | indexing ^ | exponentiation (right to left) - + | unary minus and plus : | sequence operator %any% | special operators (including %% and %/%) * / | multiply, divide + - | (binary) add, subtract < > <= >= == != | ordering and comparison ! | negation & && | and | || | or ~ | as in formulae -> ->> | rightwards assignment <- <<- | assignment (right to left) = | assignment (right to left) ? | help (unary and binary) --- class: sectionTitle # Introspection & Coercion --- # Introspection In `R`, each variable can be queried about it's `class` (what kind of data that particular variable holds). ```r x <- 42 class( x ) ``` ``` ## [1] "numeric" ``` -- You can also ask if it is a particular type using the `is.numeric()` function. ```r is.numeric( x ) ``` ``` ## [1] TRUE ``` --- # Coercion We can also turn *one representation* of our data into a different different type, though there are limitations. For example, if we just read in a text file and it has a represented as text (a [Character Data Type](../character_data/slides.html) in `R`) but we need to have it function as a `numeric` type, we can use the following approach ```r x <- "42" class( x ) ``` ``` ## [1] "character" ``` -- The create a new variable who (if possible) contains the numeric representation of the character string `"42"`. ```r y <- as.numeric( x ) class(y) ``` ``` ## [1] "numeric" ``` --- # Coercion Fail When it fails, it returns a warning and a missing data value. ```r as.numeric( "Bob" ) ``` ``` ## Warning: NAs introduced by coercion ``` ``` ## [1] NA ``` -- <div class="box-red">It should be noted that error messages in R may not be "comprehensible" to the user (and maybe not even to the programmer).</div> --- class: sectionTitle # Caveats --- # Order of Operations There are times that the order of operations will really come back to .red[bite you]. Consider this example where I create a sequence of numbers using the sequence operator (`:`) ```r n <- 4 1:n ``` ``` ## [1] 1 2 3 4 ``` -- So if we wanted to make a sequence from 1 to `\(n-1\)`, we *could* type this: ```r 1:n-1 ``` -- ``` ## [1] 0 1 2 3 ``` --- To *fix* this, feel free to be *verbose* in your use of parentheses. If you are intending to get `\(10^2\)`, `\(10^3\)` `\(\ldots\)` `\(10^6\)` and type it as: ```r 10^2:6 ``` ``` ## [1] 100 99 98 97 96 95 94 93 92 91 90 89 88 87 86 85 84 83 82 ## [20] 81 80 79 78 77 76 75 74 73 72 71 70 69 68 67 66 65 64 63 ## [39] 62 61 60 59 58 57 56 55 54 53 52 51 50 49 48 47 46 45 44 ## [58] 43 42 41 40 39 38 37 36 35 34 33 32 31 30 29 28 27 26 25 ## [77] 24 23 22 21 20 19 18 17 16 15 14 13 12 11 10 9 8 7 6 ``` -- What you want is: ```r 10^(2:6) ``` ``` ## [1] 1e+02 1e+03 1e+04 1e+05 1e+06 ``` <div class="box-yellow">Notice how the second (and intended) code is actually easier to read than the first.</div> --- # Numerical Approximations Computers use binary switches to represent numbers. For integers, it is great, but for floating point numbers it .red[sucks], big time. Consider the following: ```r x <- .1 y <- .3 / 3 ``` But if we ask if they are equal, what do you expect? -- ```r x == y ``` ``` ## [1] FALSE ``` -- ```r print(x, digits=20) ``` ``` ## [1] 0.10000000000000000555 ``` ```r print(y, digits=20) ``` ``` ## [1] 0.099999999999999991673 ``` --- class: middle background-image: url("images/contour.png") background-position: right background-size: auto .center[ # Questions? 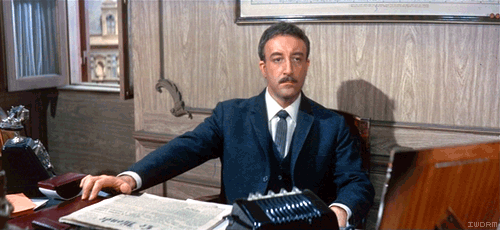 ] <p> </p> .bottom[ If you have any questions for about the content presented herein, please feel free to [submit them to me](https://docs.google.com/forms/d/e/1FAIpQLScrAGM5Zl8vZTPqV8DVSnSrf_5enypyp0717jG4PZiTlVHDjQ/viewform?usp=sf_link) and I'll get back to you as soon as possible.]